How to Use AWS Parameter Store with PHP Code Examples
Here’s a step-by-step overview of how to use AWS Parameter Store with PHP.
- Create an AWS account.
- Install AWS SDK for PHP.
- Create a
SsmClient
object. - Create a parameter using
putParameter()
. - Retrieve parameters from the store using
getParameter(
). - Update a parameter using
putParameter()
with the Overwrite argument. - Delete a parameter using
deleteParameter()
.
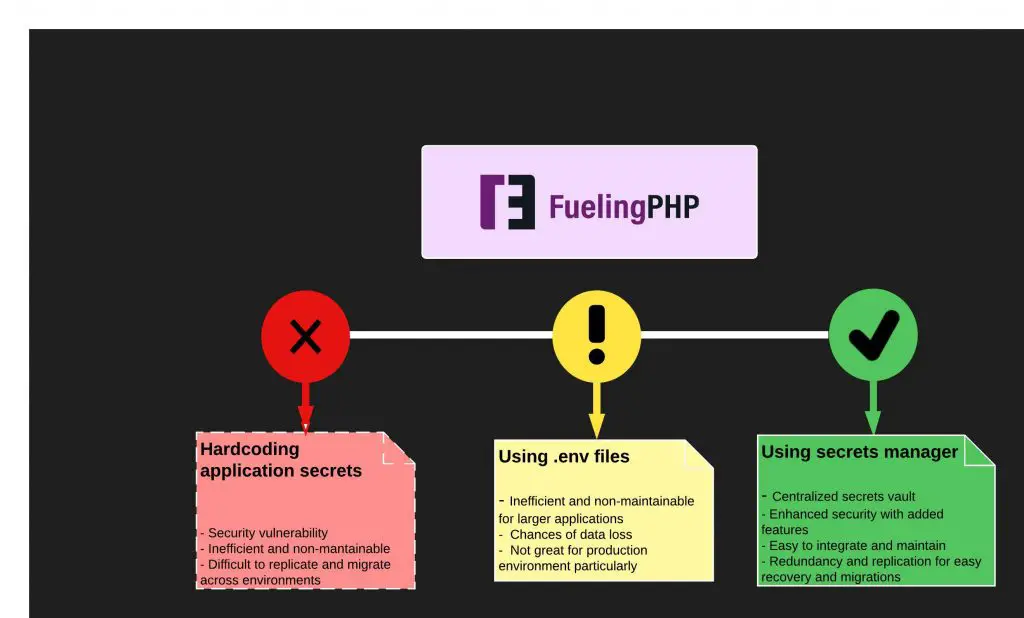
function getParameters($client, $path, $decrypt = true) {
try {
$result = $client->getParametersByPath([
"Path" => $path,
"WithDecryption" => $decrypt
]);
return $result;
} catch(AwsException $e) {
print_r('Error: ' . $e->getAwsErrorMessage());
}
}
Free AWS Development Guide

Stop running in circles and develop your applications faster and cheaper in AWS. This guide will walk you through ways to maximize AWS to generate real value for your needs. We pick the right services to scale, tighten security and maximize costs.
Download our free guide now and get started with confidence.
Dig Deeper into Managing Secrets & Environment Variables in PHP Applications
- How to use AWS Secrets Manager with PHP Code Examples – PHP Env 101
- How to Install AWS SDK for PHP – Complete Guide
- Host and Deploy a Laravel PHP App to AWS Elastic Beanstalk
Article Assumptions Regarding AWS Param Store
The article assumes the following.
- You have an AWS account and can log in to the console.
- You have installed SDK for PHP.
When should I use AWS Secrets Manager vs Param Store?
Ever heard of AWS Secrets Manager? It is yet another AWS secret vault service, but it has added benefits. The article includes a comparison of the two. FuelingPHP also features a detailed article on AWS Secrets Manager.
Hopefully, these resources will help you pick the right service for your project.
Keeping your Application’s Secrets safe in PHP with AWS
Applications usually include credentials, API keys, tokens and similar secrets that are not meant to be shared or exposed. They are absolutely necessary to integrate tools like databases and caches as well as third-party services like Email and SMS. So, what options are available to use these secrets safely and securely?

Hardcoding secrets? It may sound convenient, but it is the most naive and dangerous thing to give away secrets in your application code. It exposes your application to vulnerabilities and cyber attacks. So, it is a big NO!
Using .env files? Yes, sometimes a .env file may be a swift workaround, but it is not the best way, especially when the application scales up. These .env files are difficult to maintain, recover and replicate across environments. It is certainly not a good choice for production environments.

Using a secrets manager? A secrets vault or a manager is a service that, at the bare minimum, let’s you store the secrets in a centralized location and opens up APIs to access these secrets in your codebase. AWS Secrets Manager and Parameters Store are prime examples. (Though secrets manager has some additional features – but more on that later!)
A secret manager is easier to replicate, recover and maintain. Besides, services like AWS Secrets Manager offers encryption, rotation and monitoring of secrets. So, it is definitely worth it to use these for your application to ensure more security and consistency.
We have just mentioned two services: AWS Secrets Manager & Parameter Store. FuelingPHP features a detailed article on AWS Secrets Manager. This article focuses on AWS Parameter Store. If you’re not sure which to use in your project, jump to the comparison section, as it will assist you in picking the right service for your project.
What is AWS Parameter Store?
AWS Parameter Store is a service that lets you store application secrets securely in one place in a defined hierarchy. It falls under the umbrella of a vast service called AWS Systems Manager, an end-to-end management solution for AWS and on-premises resources. Though the Systems Manager features four core groups of management, the Parameter Store falls in the “Application Management” group.

AWS Parameters store uses the standard tier (by default), but you can always switch to the advanced tier. Standard-tier uses 4KB as the max size for a parameter and doesn’t allow parameter policies. The advanced tier uses an 8KB as the max size for a parameter and allows parameter policies – of course, at a cost.
Tier | Price |
Standard (Default) | No extra cost |
Advanced | $0.05 per advanced parameter per month |
On top of that, the API incurs charges as follows.
Tier | Standard throughput | High throughput |
Standard (Default) | No extra cost | $0.05 per 10,000 API interactions |
Advanced | $0.05 per 10,000 API interactions | $0.05 per 10,000 API interactions |
AWS features intelligent tiering for Parameter Store that lets the Store decide if the incoming parameter should be standard or advanced.
A Comparison | AWS Secrets Manager vs Parameter Store
To make a meaningful comparison, you should be aware of the AWS Secrets Manager.
Similarities | AWS Secrets Manager vs Parameter Store
Managed secrets vault services
Both Secrets Manager and Parameter Store are managed by secret vault services at their base. Secrets Manager offers some extra features that add more to the security, but the base proposition is the same. You can use them for storing application secrets in a key/value format. In addition, Parameter Store can integrate with the Secrets Manager, and the former can retrieve secrets from the latter. Learn more about how Parameter Store can refer to the secrets in the Secrets Manager.
Encryption
Both services use AWS KMS to encrypt data. Parameter Store is flexible in choosing whether the secrets should be encrypted. On the contrary, AWS Secret Manager does encryption and leaves no option for unencrypted data.
Besides, both services use AWS IAM for granular access control to these resources. So, only authorized users can access the vaults.
Differences | AWS Secrets Manager vs Parameter Store
Secrets rotation
AWS Secret Manager features native auto credential rotation for AWS RDS. For other services, it uses AWS Lambda functions. Rotating a credential at regular intervals is an intelligent security practice. The cool thing is that your application remains largely unaware of these changes because it is all done by the Secrets Manager.
On the other hand, Parameters Store doesn’t support rotation natively yet.
Cost
The standard tier Parameter Store doesn’t charge for storage. It does charge $0.05 for 10,000 API interactions, but that sounds minimal. AWS Secrets Manager costs $0.40 per secret and $0.05 for 10,000 API interactions. So, if you are about to store a lot of secrets, keep this cost estimate in your mind.
Pros & Cons of using AWS Parameter Store in PHP
Pros | Cons |
Cost efficient | No native support for automatic credentials rotation |
Simple, bare-bones secret vault. | |
Features encryption using KMS | |
Integrate with other AWS services like Secrets Manager, Cloudformation, Cloudtrail, SNS, EventBridge and KMS | |
Accessible from various AWS services like EC2, AWS Lambda, ECS, Cloudbuild and others | |
Out-of-the-box data validation for AWS EC2 AMI resource string format | |
Uses AWS IAM for fine granular access control |
Features of AWS Parameter Store
Safety & security
AWS Parameter store features SafetyString types. These are not ordinary string types but come with encryption via AWS KMS. On top of encryption at rest, this service allows fine-grain access control using AWS IAM.
Though it doesn’t feature automatic rotation like AWS Secrets Manager, it still gives alot to securely store your credentials using hierarchical paths to ensure uniqueness for every parameter.
Note: Using AWS KMS custom keys will add the current KMS rate to the base costs. Learn more about KMS pricing.
Integration with other AWS services
Another useful feature is that it integrates well with other AWS services for various functionalities, including access control, encryption, monitoring and auditing. These include CloudWatch, Cloudtrail, IAM, KMS and Secret Manager itself. Infact, it can refer to the secrets in the secrets manager vault.
The integration goes two ways: parameters are accessible by other AWS services including EC2, ECS, AWS Lambda, CloudFormation, CloudPipeline, CodeBuild & CodeDeploy.
Data Validation
The parameter store can validate data that refer to the Amazon EC2 instance. Usually, these strings point to an EC2 resource in your AWS account. These are AMIs, and they follow a certain format. The parameter store can validate these strings and also ensure that the said resource exists.
Efficient Client API
AWS SDK for PHP features an SSMCliet that allows access to many different methods, including those used throughout this article. Your program needs to reference an SSMClient; the rest is just a call. You can access parameters anywhere in your code via these functions.
Use AWS Parameter Store via AWS Console
Create a parameter store
Sign In to the AWS console and navigate AWS Systems Manager.

In the Systems Manager, navigate to the Parameter Store and click Explore Parameter Store.

In the Parameter Store, click Create parameter.

In the Create Parameter, provide the following.
- Name for the parameter store. You can define unique hierarchies. For example, /dev/fuelingphp/apikey or /prod/fuelingphp/apikey.
- Description for the parameter store is optional but good to have.
- Type refers to the data type. AWS Parameter Store expects any of these three types.
- String is any ordinary textual value. For instance – Hello world
- StringList is a list of texts separated by commas. For instance – one, two, three.
- SecureString encrypts the parameters using KMS. Ideal for storing credentials.
- KMS key source means the encryption key you want to use. Use the default KMS key if you don’t have a custom key. Note that custom keys can incur extra costs.
- Provide a value you want to store. For instance, FuelingPHP-API-KEY.
Once you’re good to go with these, click Create parameter.

If everything goes well, the parameter comes to life in the parameter store.

Retrieve a parameter from the parameter store
Click on the parameter store just created above, and it opens up the “dev/fuelingphp/apikey” parameter.
Click on the show option to decrypt and reveal the secret.

Edit parameter from the parameter store
Click on the Edit button to make changes to the parameter. Let’s change the value to “api-key-fuelingphp”. Click Save when done.

Note that the version changes from 1 to 2.
Delete parameter from the parameter store
Click on the Delete button to make changes to the parameter.

The parameter will be removed readily.
Use AWS Parameter Store with PHP Code via AWS SDK Example.
How to Create SSMClient in PHP Code
AWS SDK features the SSMClient object, an API to interact with the AWS Parameter store using PHP.
<?php
require "vendor/autoload.php";
use Aws\Ssm\SsmClient;
$parameterStoreClient = new SsmClient([
'profile' => 'default',
'region' => 'us-east-1',
'version' => "latest"
]);
?>
The SSMClient expects an AWS profile with valid permissions. We pass the default profile that has been a while ago. Creating an AWS profile for programmatic access is out of the scope of this tutorial, but you can learn how to create one here.
Create a parameter in the AWS parameter store with PHP
Let’s create a new vault via PHP with the path “/dev/fuelingphp/apikey”.
The SsmrClient object feature putParameter() will help us create a parameter. It expects the same arguments we have seen while creating a parameter in the console. We will provide the name and value.
function putParameter($client, $configArray) {
try {
$result = $client->putParameter([
"Name" => isset($configArray["Name"]) ? $configArray["Name"] : "/dev/fuelingphp/default",
"Description" => isset($configArray["Description"]) ? $configArray["Description"] : "No description",
"Type" => isset($configArray["Type"]) ? $configArray["Type"] : "SecureString",
"Value" => isset($configArray["Value"]) ? $configArray["Value"] : "default",
"Overwrite" => isset($configArray["Overwrite"]) ? $configArray["Overwrite"] : false
]);
return $result;
} catch(AwsException $e) {
print_r('Error: ' . $e->getAwsErrorMessage());
}
}
Calling this function creates a new parameter and returns an object with the version number of the parameter.
Retrieve AWS parameters from the store with PHP Example
We have created a wrapper function that expects an SSMClient object, a path and an optional decrypt argument which defaults to true. It calls the getParametesrByPath() method on the client object.
A path should refer to the (n-1) level in the path hierarchy, which means that if we have a path “/dev/fuelingphp/apikey” we should pass “/dev/fuelingphp” only. Many parameters can be grouped in the “/dev/fuelingphp” hierarchy.
function getParameters($client, $path, $decrypt = true) {
try {
$result = $client->getParametersByPath([
"Path" => $path,
"WithDecryption" => $decrypt
]);
return $result;
} catch(AwsException $e) {
print_r('Error: ' . $e->getAwsErrorMessage());
}
}
This method returns a response object if everything goes well.
$parameters = getParameters($parameterStoreClient, "/dev/fuelingphp");
echo json_encode($parameters["Parameters"], JSON_PRETTY_PRINT);
The parameter data in the JSON looks as follows.
[
{
"Name": "\/dev\/fuelingphp\/apikey",
"Type": "SecureString",
"Value": "api-key-fuelingphp",
"Version": 1,
"LastModifiedDate": "2022-12-01T20:58:11+00:00",
"ARN": "arn:aws:ssm:us-east-1:515090671654:parameter\/dev\/fuelingphp\/apikey",
"DataType": "text"
}
]
Edit AWS parameters from the store with PHP Example
We can reuse the putParameter() with overwrite argument set to true. This way, we can rewrite an existing parameter that could change its value and version.
Let’s update “api-key-fuelingphp” to “api-key-fuelingphp-new”
$updatedParameter = putParameter($parameterStoreClient, ["Name" => "/dev/fuelingphp/apikey", "Value"=>"api-key-fuelingphp-new", "Overwrite" => true]);
The output returns an object with the success status code, version and other metadata.
Delete AWS parameters from the store with PHP Example
The function deleteParameter() expects a client object and a fully-qualified parameter name for deletion.
function deleteParameter($client, $name) {
try {
$result = $client->deleteParameter([
"Name" => $name,
]);
return $result;
} catch(AwsException $e) {
print_r('Error: ' . $e->getAwsErrorMessage());
}
}
Voila! That’s a lot of ground to cover. Let’s wrap up this article. See you next time!
How to Use AWS Param Store in my PHP App
This article demonstrates how to use AWS Parameter Store in a PHP application. You can integrate the AWS via the composer SDK that opens up the param store APIs for your PHP app. Integrate Param Store in your pipelines and configurations for best results.
We use the AWS console to create a store and put parameters in it so that users who prefer a more GUI-based approach can easily find their way through this service. Apart from that, the article opens up with some insights about the AWS Parameter Store itself, including its pricing model, features, pros, and cons.
The article also compares this service to AWS Secrets Manager to educate readers about the utility of both of these and ultimately help them pick the right service for their project.
Free AWS Development Guide

Stop running in circles and develop your applications faster and cheaper in AWS. This guide will walk you through ways to maximize AWS to generate real value for your needs. We pick the right services to scale, tighten security and maximize costs.
Download our free guide now and get started with confidence.
Building Web Applications in AWS
This article is part of our series to make AWS easy. We love AWS, but let’s be honest. It isn’t effortless. It’s way too complicated. We’ve created this learning path to help level you up and onboard your PHP app quickly.
- Is AWS Cloud Services Hard to Learn?
- Why AWS is so Popular
- AWS PHP Website & Application Hosting Options
- Using PHP in AWS Lambda: Comparing Options
- Deploy Laravel to Elastic Beanstalk using GitHub Actions
- Deploy Laravel from Github to Elastic Beanstalk via CodePipeline
- Install LAMP Stack on AWS EC2
- Install AWS SDK for PHP
- Host and Deploy a Laravel PHP App to AWS Elastic Beanstalk
- Amplify vs. Elastic Beanstalk
- AWS App Runner Review
- App Runner vs Elastic Beanstalk
- App Runner vs. Fargate
- Elastic Beanstalk vs AWS Lightsail
- Elastic Container Service vs. Beanstalk
- Setup & Use AWS Parameter Store in PHP
- How to use AWS Secrets Manager with PHP
- AWS Equivalent of Azure Service Bus
- PHP & AWS S3